Basic Shapes¶
IPKISS shapes are all subclasses of the basic Shape
class.
Basic shape |
|
Basic circle |
|
Circular arc |
|
Circular arc specified by its starting point instead of its center |
|
Cross. |
|
Wedge, or symmetric trapezium. |
|
Radial wedge: the coordinates of the start and end point are specified in polar coordinates from a given center |
|
Basic ellipse |
|
Ellipse arc around a given center. |
|
Basic rectangle |
|
Rectangle with rounded corners |
|
Ring segment |
|
Regular N-sided polygon |
|
Hexagon |
|
Dodecagon |
|
Parabolic wedge (taper) |
|
Exponential wedge (taper) |
Shape¶
- class ipkiss3.all.Shape(points=[], closed=None, **kwargs)¶
Basic shape
- Parameters
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
>>> shape = Shape(points=[(0.0, 0.0), (10.0, 10.0)]) >>> shape.points # returns the points of the shape >>> array([[ 0., 0.], >>> [ 10., 10.]])
>>> shape.get_face_angles() # returns (start_face_angle, end_face_angle), >>> (45.0, 45.0) # these are the angles at the start and the end of an open shape.
>>> shape.closed # returns whether the shape is closed or not. >>> False
>>> wg_layout = i3.Waveguide().Layout(shape=shape) >>> wg_layout.visualize()
The start_face_angle is not equivalent to the waveguide port angle, as it points inwards and not outwards. The end_face_angle, however, is equivalent to the waveguide port angle. >>> shape.start_face_angle = 10. >>> wg_layout2 = i3.Waveguide().Layout(shape=shape) >>> wg_layout2.visualize()
Examples
import ipkiss3.all as i3
import matplotlib.pyplot as plt
s1 = i3.Shape([(5.0, -5.0), (0.0, 0.0), (5.0, 5.0), (10.0, 0.0)], closed=False)
p2 = i3.Coord2((15.0, 0.0))
s2 = s1 + p2
plt.figure()
plt.plot(s2.x_coords(), s2.y_coords(), 'bo-', markersize=8, linewidth=2)
plt.show()
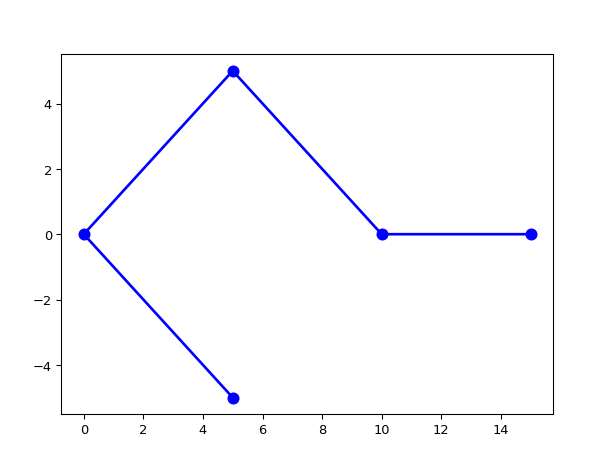
ShapeCircle¶
- class ipkiss3.all.ShapeCircle(**kwargs)¶
Basic circle
- Parameters
- box_size: optional
- radius: float and number > 0, optional
radius of the circular arc
- end_angle: optional
- start_angle: optional
- angle_step: float, optional
discretization angle
- center: Coord2, optional
center of the ellipse
- clockwise: ( bool, bool_, bool or int ), optional
orientation of the arc. clockwise:True
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
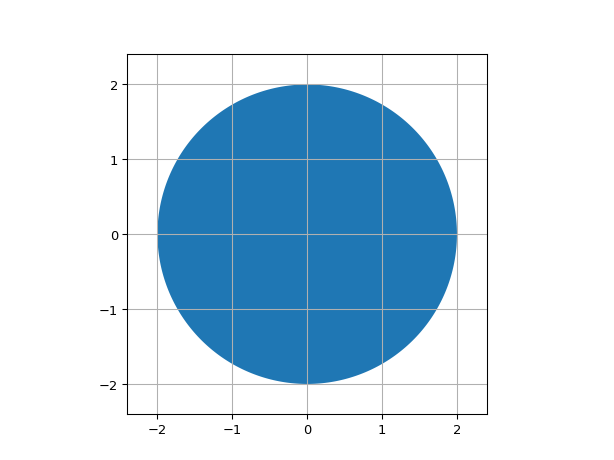
ShapeArc¶
- class ipkiss3.all.ShapeArc(**kwargs)¶
Circular arc
- Parameters
- box_size: optional
- radius: float and number > 0, optional
radius of the circular arc
- angle_step: float, optional
discretization angle
- center: Coord2, optional
center of the ellipse
- clockwise: ( bool, bool_, bool or int ), optional
orientation of the arc. clockwise:True
- end_angle: float, optional
end angle of the arc according to the parametric representation of an ellipse
- start_angle: float, optional
start angle of the arc according to the parametric representation of an ellipse
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
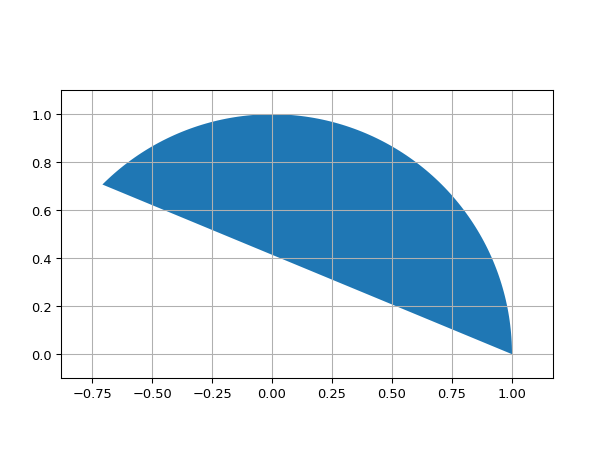
ShapeBend¶
- class ipkiss3.all.ShapeBend(**kwargs)¶
Circular arc specified by its starting point instead of its center
- Parameters
- center: optional
- end_angle: optional
- input_angle: float, optional
- output_angle: float, optional
- start_angle: optional
- start_point: Coord2, optional
starting point of the circular bend
- box_size: optional
- radius: float and number > 0, optional
radius of the circular arc
- angle_step: float, optional
discretization angle
- clockwise: ( bool, bool_, bool or int ), optional
orientation of the arc. clockwise:True
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
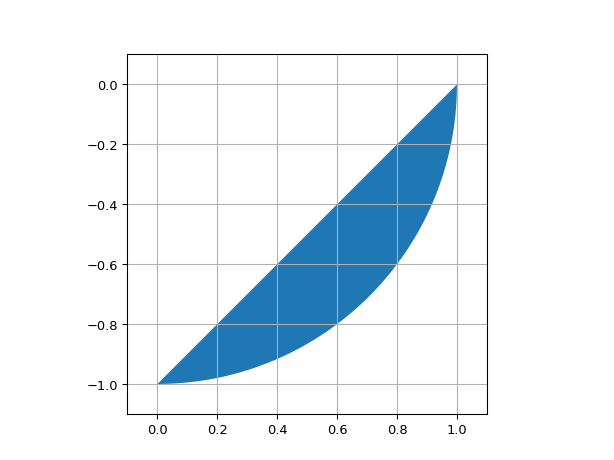
ShapeCross¶
- class ipkiss3.all.ShapeCross(**kwargs)¶
Cross. Thickness sets the width of the arms
- Parameters
- box_size: float and number > 0, optional
- center: Coord2, optional
- thickness: float and number > 0, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
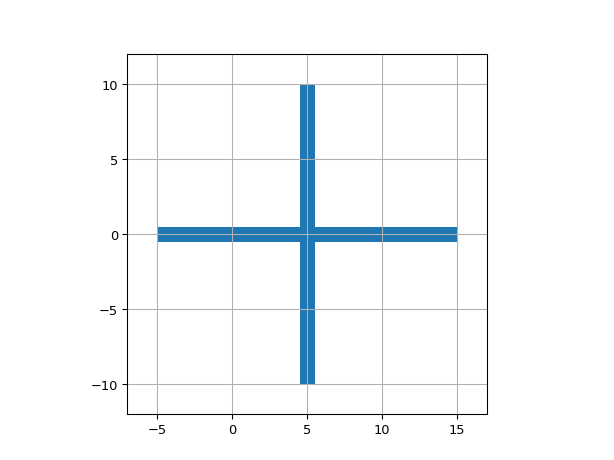
ShapeWedge¶
- class ipkiss3.all.ShapeWedge(**kwargs)¶
Wedge, or symmetric trapezium. Specified by the center of baselines and the length of the baselines
- Parameters
- begin_coord: Coord2, optional
- begin_width: float and Real, number and number >= 0, optional
- end_coord: Coord2, optional
- end_width: float and Real, number and number >= 0, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
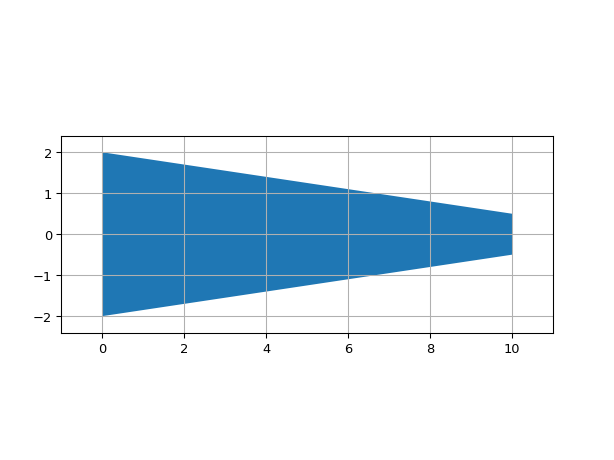
ShapeRadialWedge¶
- class ipkiss3.all.ShapeRadialWedge(**kwargs)¶
Radial wedge: the coordinates of the start and end point are specified in polar coordinates from a given center
- Parameters
- angle: float, required
- inner_radius: float and number > 0, required
- inner_width: float and number > 0, required
- outer_radius: float and number > 0, required
- outer_width: float and number > 0, required
- center: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
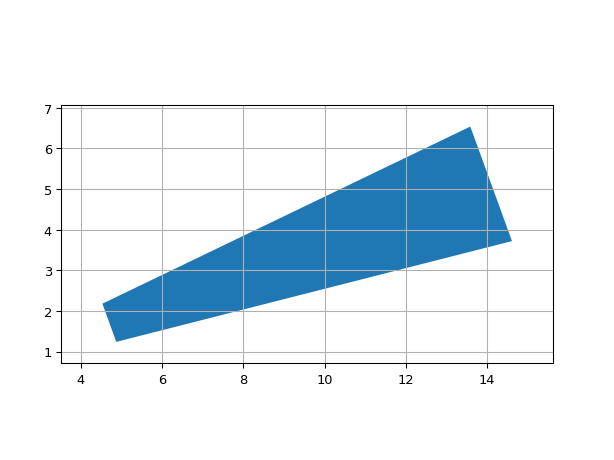
ShapeEllipse¶
- class ipkiss3.all.ShapeEllipse(**kwargs)¶
Basic ellipse
- Parameters
- end_angle: optional
- start_angle: optional
- angle_step: float, optional
discretization angle
- box_size: Coord2 and number >= 0, optional
size of the ellipse along major and minor axis
- center: Coord2, optional
center of the ellipse
- clockwise: ( bool, bool_, bool or int ), optional
orientation of the arc. clockwise:True
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
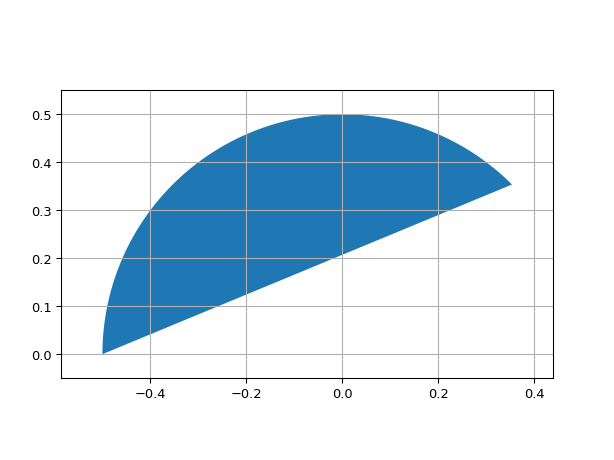
ShapeEllipseArc¶
- class ipkiss3.all.ShapeEllipseArc(**kwargs)¶
Ellipse arc around a given center.
ShapeEllipseArc implements the standard parametric representation of an ellipse (x = a * cos(t), y = b * sin(t)) and (0 <= t < 2 * pi), where parameter t is not the actual angle, but has a geometric meaning due to Philippe de La Hire.
The start_angle and end_angle are described as this parameter t.
- Parameters
- angle_step: float, optional
discretization angle
- box_size: Coord2 and number >= 0, optional
size of the ellipse along major and minor axis
- center: Coord2, optional
center of the ellipse
- clockwise: ( bool, bool_, bool or int ), optional
orientation of the arc. clockwise:True
- end_angle: float, optional
end angle of the arc according to the parametric representation of an ellipse
- start_angle: float, optional
start angle of the arc according to the parametric representation of an ellipse
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Notes
If you want to get the parametric angle t from the actual angle alpha, you can use the following:
import numpy as np a, b = box_size t = np.arctan2(2. / b * np.sin(alpha), 2. / a * np.cos(alpha)) # t and alpha in radians
Examples
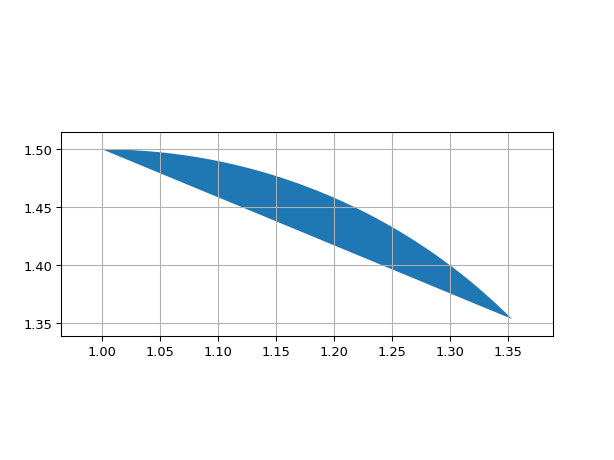
ShapeRectangle¶
- class ipkiss3.all.ShapeRectangle(**kwargs)¶
Basic rectangle
- Parameters
- radius: optional
- angle_step: float, optional
angle_step using in the rounding.
- box_size: Coord2 and number >= 0, optional
size of the rectangle.
- center: Coord2, optional
center of the rectangle.
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
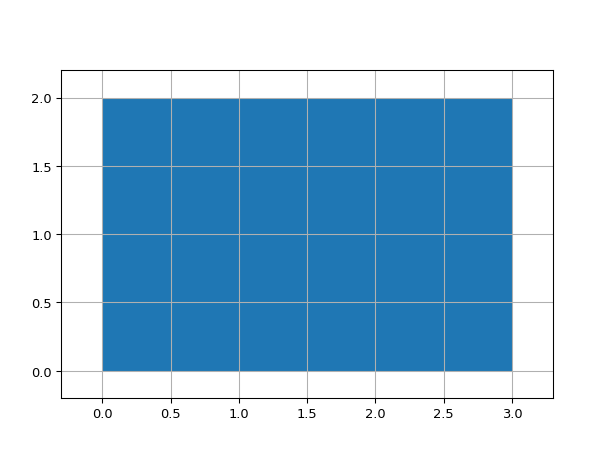
ShaperoundedRectangle¶
- class ipkiss3.all.ShapeRoundedRectangle(**kwargs)¶
Rectangle with rounded corners
- Parameters
- angle_step: float, optional
angle_step using in the rounding.
- box_size: Coord2 and number >= 0, optional
size of the rectangle.
- center: Coord2, optional
center of the rectangle.
- radius: float and Real, number and number >= 0, optional
radius of the rounding used.
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
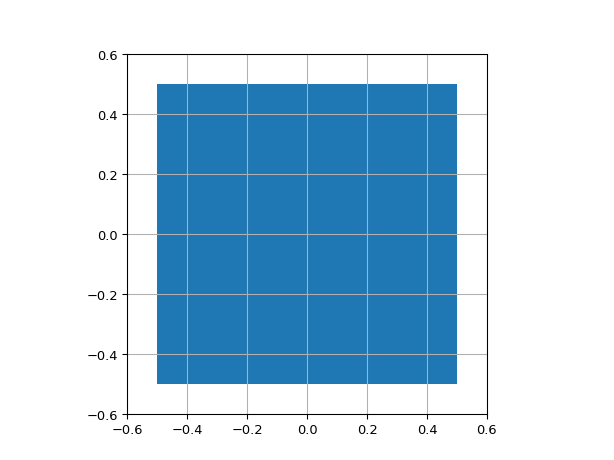
ShaperingSegment¶
- class ipkiss3.all.ShapeRingSegment(**kwargs)¶
Ring segment
- Parameters
- inner_radius: float and number > 0, required
- outer_radius: float and number > 0, required
- angle_end: float, optional
- angle_start: float, optional
- angle_step: float, optional
- center: Coord2, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
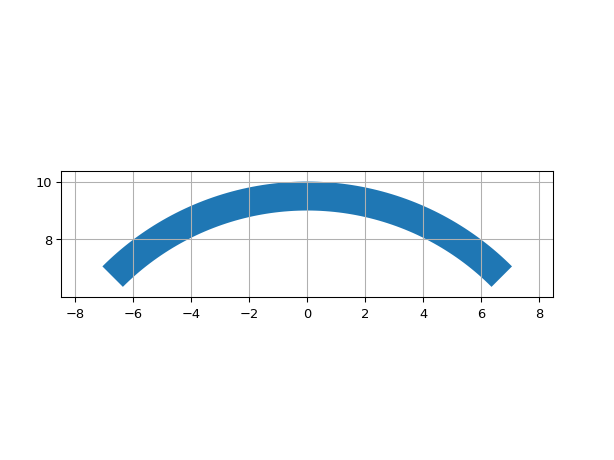
ShapeHexagon¶
- class ipkiss3.all.ShapeHexagon(**kwargs)¶
Hexagon
- Parameters
- center: Coord2, optional
Center of the polygon
- radius: float and number > 0, optional
Radius of the polygon
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- n_o_sides: int and [3,None], locked
Number of sides of the hexagon
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
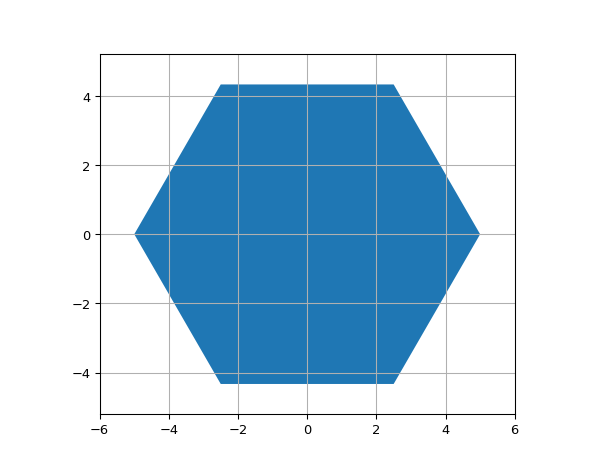
ShapeDodecagon¶
- class ipkiss3.all.ShapeDodecagon(**kwargs)¶
Dodecagon
- Parameters
- n_o_sides: int and [3,None], optional
- center: Coord2, optional
Center of the polygon
- radius: float and number > 0, optional
Radius of the polygon
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
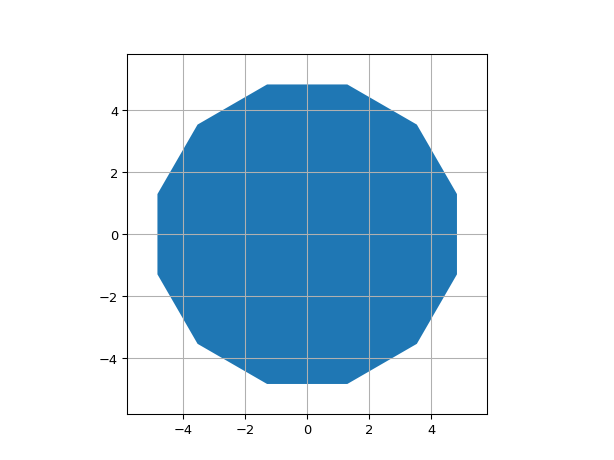
ShapeRegularPolygon¶
- class ipkiss3.all.ShapeRegularPolygon(**kwargs)¶
Regular N-sided polygon
- Parameters
- center: Coord2, optional
Center of the polygon
- n_o_sides: int and [3,None], optional
Number of sides fo the polygon
- radius: float and number > 0, optional
Radius of the polygon
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
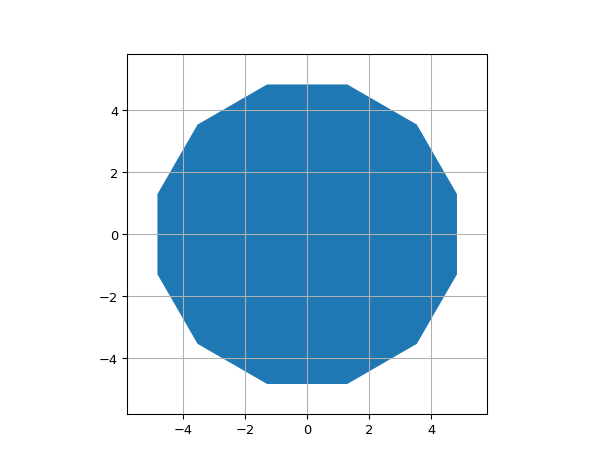
ShapeParabolic¶
- class ipkiss3.all.ShapeParabolic(**kwargs)¶
Parabolic wedge (taper)
- Parameters
- begin_coord: Coord2, optional
- begin_width: float and Real, number and number >= 0, optional
- end_coord: Coord2, optional
- end_width: float and Real, number and number >= 0, optional
- width_step: float and number > 0, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
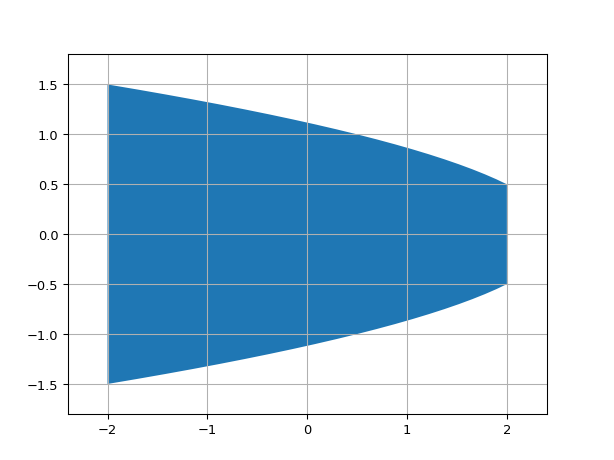
ShapeExponential¶
- class ipkiss3.all.ShapeExponential(**kwargs)¶
Exponential wedge (taper)
- Parameters
- begin_coord: Coord2, optional
- begin_width: float and Real, number and number >= 0, optional
- end_coord: Coord2, optional
- end_width: float and Real, number and number >= 0, optional
- g: float and Real, number and number >= 0, optional
Exponential growth constant. Defaults to ln(max_width/min_width)
- width_step: float and number > 0, optional
- closed: optional
- end_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the end of an open shape
- points: optional
points of this shape
- start_face_angle: ( float ), optional, *None allowed*
Use this to overrule the ‘dangling’ angle at the start of an open shape
- Other Parameters
- size_info: SizeInfo, locked
get the size information on this Shape
Examples
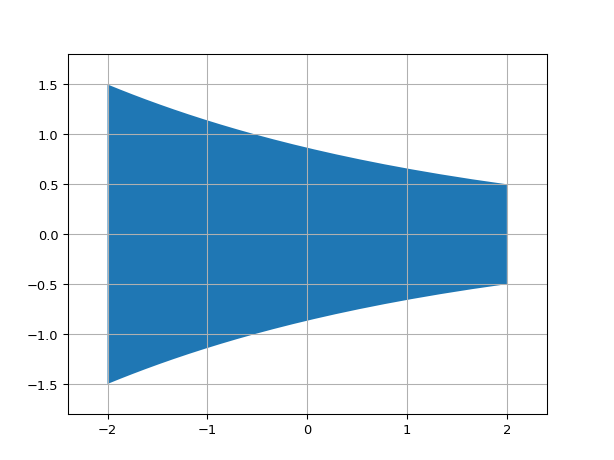